Hello,
I have a very simple grid graph set up in my scene, with 1 enemy, 1 player, and a few areas that I want to set up as Hazardous or Difficult on a per-agent basis with tags. As a very simple test, I added two Unity tags (Difficult and Hazardous). I added the same tags in A* Settings. I altered the grid graph to add a per layer modification, mapping the unity layers to the A* layers. I scan the graph, using debug show tags, looks great.
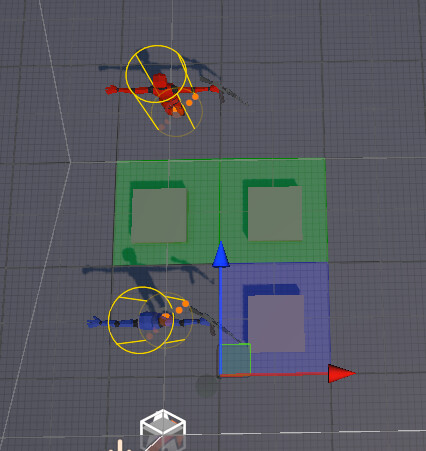
Jumping over to my agent, who is using AIPath. I jump into the seeker and set the tags to be not traversable (for testing), and add a penalty for testing and debugging.
The agent is still calculating a path that goes right through them (i get path cost as 2350 , it should be 12000 … each grid square is 2 units and I understand 1 unit = 10000 cost).
I am not sure if this is because I am manually calculating the path (I am doing a multitarget path from the enemy to every adjacent square of the player) or what is going wrong here.
Do you have the code for how you are calculating the path?
Yup! You were faster than me 
I thought it was because of how I am constructing the path (since I don’t use the agent in the construction of the path) , but then was confused because this specific seekers penalties are being applied to the product. I couldn’t figure out how to pass this seekers information into the path construction.
The construction of the path is very dirty, please forgive my ugly learning code 
First I gather the players and create a pathfinding unit — this uses constantpath to get adjacent nodes.
private class PathfindingUnit
{
public Unit Unit { get; private set; }
public List<PathfindingPosition> positions;
public PathfindingPosition leastCost;
public PathfindingUnit(Unit unit)
{
Unit = unit;
var path = ConstantPath.Construct(Unit.transform.position, (1 * 2 + 1) * 1000);
AstarPath.StartPath(path);
path.BlockUntilCalculated();
var allNodes = path.allNodes;
allNodes.Remove(path.startNode);
var allValidNodes = allNodes.FindAll(node =>
Unit.LevelGrid.IsValidGridPosition((Vector3)node.position) &&
!Unit.LevelGrid.IsGridPositionOccupied(
Unit.LevelGrid.GetGridPositionFromWorld((Vector3)node.position)));
positions = new();
foreach (var graphNode in allValidNodes)
{
PathfindingPosition pathfindingPosition = new PathfindingPosition();
pathfindingPosition.worldPos = (Vector3)graphNode.position;
pathfindingPosition.gridPosition = Unit.LevelGrid.GetGridPositionFromWorld((Vector3)graphNode.position);
pathfindingPosition.cost = int.MaxValue;
positions.Add(pathfindingPosition);
}
}
public void CalcCost(Vector3 startPos)
{
MultiTargetPath p = MultiTargetPath.Construct(startPos,
positions.Select(pos => pos.worldPos).ToArray(),
null, null);
p.pathsForAll = true;
AstarPath.StartPath(p);
p.BlockUntilCalculated();
for (int i = 0; i < p.nodePaths.Length; i++)
{
// if (p.targetPathCosts[i] == null)
// positions[i].cost = uint.MaxValue;
positions[i].cost = p.targetPathCosts[i];
}
positions.Sort((obj1, obj2) => obj1.cost.CompareTo(obj2.cost));
leastCost = positions[0];
}
}
Right after creating the unit CalcCost is called. When I say the path cost is 2350 it is from A*'s debugging, not from looking at the leastCost stored in my classes
Hi
It looks like you not using the Seeker to start the path. In that case, it cannot apply its settings.
The Seeker has a StartMultiTargetPath method, if you want to use it.
Otherwise, you can copy the info, before you call AstarPath.StartPath.
path.enabledTags = seeker.traversableTags;
path.tagPenalties = seeker.tagPenalties;
furiously testing will let you know how it goes, that link may drastically reduce the complexity and computational cost of what I am trying to do here too lol, thanks! Hopefully it all goes well
I hope your recovery is swift ^ _ ^
1 Like