I am using the following code (that I wrote for playmaker), to path a random destination point within a range. I am using the seeker component and AI Path component on a game object for movement.
Often the agent gets stuck before reaching the destination. It seems like maybe the final destination point is in an unwalkable zone? Can you check my random path script to see if I am making an error somewhere? (this code was based on an example posted here).
(variable.Value, just means playmaker can access the variable
go = the current game object
).
var rndPoint = go.transform.position + Random.insideUnitSphere * Random.Range(MinWanderArea.Value, MaxWanderArea.Value);
var closestPointOnNavmesh = AstarPath.active.GetNearest(rndPoint).clampedPosition;
randomDestination.Value = closestPointOnNavmesh;
seeker.StartPath(go.transform.position, closestPointOnNavmesh, OnPathComplete);
If you can see in my images, the AI agent stops about 2 floats away from its final destination. It seems to be pathed into some obstacles.
Since the agent never reaches its destination, then I cannot fire an “arrived” event once it has done so. Therefore, the agent is stuck in limbo 
My distance to destination is calculate like so
distance = Vector3.Distance(go.transform.position, closestPointOnNavmesh);
Hi
What movement script are you using (AIPath?)? Could you post a screenshot of the settings for that component?
Using AI path with just the default settings in ver 4.0.10. Nothing modified on the script.
Make sure you zero out the difference along the Y axis. It looks like the pivot of the AI is in the center of it, not near its feet.
Try
var dir = closestPointOnNavmesh - go.transform.position;
dir.y = 0;
distance = dir.magnitude;
Ill give that a try. The pivot is set right at the feet.
Here is a shot of the pivot and the AI settings.
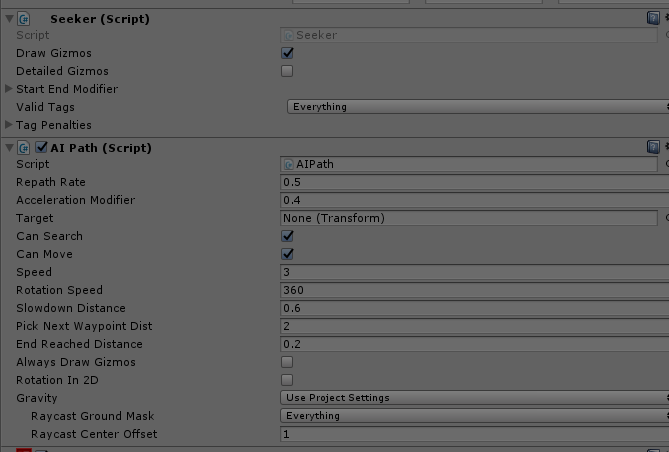
Seems to be something else. It happens maybe one out of every 3 - 5 times it paths.
Here are two examples below where the end position is NOT inside a no walk area. But the AI stopped anyways. Usually more than 1f away from the end position.
Playmaker shows the set vector3 end position. The inspector shows the current gameobject position. (Which has stopped moving)
btw. Isn’t it better to set the ‘target’ field on the AI instead of calling StartPath once? That way it will manage to avoid obstacles if any should be placed while it is traversing the path.
Also. Try to draw a line from the AI to the destination.
Debug.DrawLine(ai.transform.position, closestPointOnNavmesh, Color.magenta)
1 Like
I didnt know that actually.
I went with the seeker.StartPath, because then it could use different Path AI or custom Path AI, without having to make a playmaker action for each one.
In 4.1.0 you should be able to use GetComponent< IAstarAI >.destination = blah. This will work for AIPath, RichAI and AILerp.
1 Like
Cool.
So its defiantly trying to path into a no walk zone.
I am going to change my playmaker action into a c# script and try it on some of your example scenes to see if I get the same results.
Huh. Odd.
If you are using 4.1.0 you might want to use IAstarAI.remainingDistance by the way.
It will be more accurate that what you are using right now as well.
Note however that it is valid for the current path that the agent is traversing. If you just set the destination the new path might not have been calculated yet.
So if you start your path with
ai.destination = foo;
ai.SearchPath();
then you can check the distance with
// Make sure the path has been calculated
if (!ai.pathPending) {
if (ai.remainingDistance < bar) {
}
}
Ah. That call will find the closest node on the grid. This may be an unwalkable node however.
If you want to find the closest walkable node then you can do this:
var closestPointOnNavmesh = AstarPath.active.GetNearest(rndPoint, NNConstraint.Default).clampedPosition;
(the name ‘default’ is a bit confusing. I should change that. It is called default because that’s what paths use by default).
1 Like
I was just about done with my c# script, haha.
That sounds like it is probably the solution! Well, sorry I wasted your time, but it was illuminating for me and I got some good tips here. I am hoping the playmaker package will make it easier for noobs like me to use Astar.
My actions look like this, and have youtube tutorials to go with them.
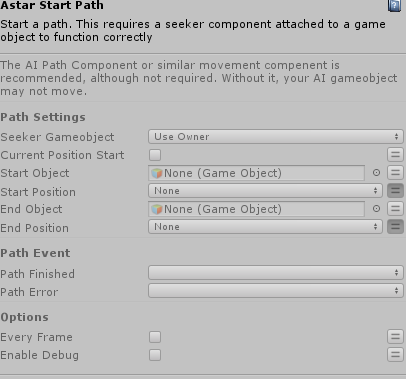
Tutorials like, point and click movement (no scripting), wandering AI who can “see”, etc. Noob stuff 
The seeker vs IAstarAI get component tip was good. Ill have to make those changes to the actions.
1 Like
While I have you here, I have a general question.
Why would one to pre-calculate a path, and then not call it right away? WHat kind of game situation?
pathToSave = ABPath.Construct (position A, postion B, null);
I wrote an action to save a path, but because playmaker cannot save A* path types, I wrote the playmaker action to save it to a dictionary file instead. I just havent actually run across a situation where I would need to save and then load a path yet.
That can be useful if you need to change settings on the path object.
When you call StartPath and you are using multithreading, a thread will start to calculate it as soon as possible, so you will want to change the settings before you start it.
Usually it is
path = ...Construct(...)
// edit settings
seeker.StartPath(path)
4.1.1.
AstarWanderAI.cs(97,92): warning CS0618: Pathfinding.NNInfo.clampedPosition' is obsolete:
This field has been renamed to ‘position’’
Just change clampedPosition to .position instead?
Sorry to hijack this thread as it is very similar to my situation here.
If my object (maybe obstacle reasons) cannot reach its destination, what is a good way to ask it to recalculate another random destination to walk to or calculate another path?
Thank you!
Vincent