Hello @aron_granberg ,
I have created a grid with characters that take more than 1 node space. As you can see in the image they know their space so they dont allow others to walk over their nodes but they don’t seem to know when constructing their available path that their size is 2x3 which therefore makes them not able to go in 1x1 corridor-like spaces.
My Traversal provider was more or less the default one from your examples but then I realized that the OverlappingNodesCost method you have is using the DefaultITraversalProvider.CanTraverse. Does that ever work? The default one isn’t checking BlockManager and I have to say it was the last piece of the puzzle for my solution.
Just curious if I was missing something or whether it’s been missed.
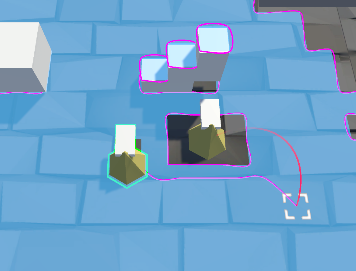
Hey there, gonna take a look at this for you and see what I can find out. Going to try and learn here with you; I haven’t used A* in this context but I’d love to try and brainstorm this together 
You referred to an example, did you mean this one? I’ll follow along with it to see what I can learn if that’s the right one.
Hi, I don’t think there’s anything to brainstorm, it’s more of a question to see if your example was tested a bit more thoroughly or whether I missed something obvious. I am referring to the one that has the OverlappingNodes method which is the GridShapeTraversalProvider.
@tealtxgr is this thread still active?
Whoops sorry about that, this one slipped past me. I’m going to replicate the example you followed so I can answer your question, but I need to know which example in the documentation you were referring to- was it this one?
I’m actually only recently joining the support team, so I’d have to follow along to see where you’re at. Let me know if that’s the correct example and I can give you a more concrete answer 
You wont find it in the examples, you need to check the one with the method name in the class i sent you earlier
Ahh, I’m seeing now. I misunderstood the first sentence of your second paragraph. I tried to do some digging to see if I could find any clues to your question, but couldn’t find any myself. I’ll ping @aron_granberg on this, and see if he has a concrete answer.
1 Like
Hi
It sounds like you want to combine custom ITraversalProvider in the turn based tutorial, with the custom ITraversalProvider in the large characters tutorial.
This is doable. You just need to call one of them from the other:
You’d replace it with something like this:
class GridShapeTraversalProvider : ITraversalProvider {
Int2[] shape;
ITraversalProvider innerProvider;
public static GridShapeTraversalProvider SquareShape (int width) {
if ((width % 2) != 1) throw new System.ArgumentException("only odd widths are supported");
var shape = new GridShapeTraversalProvider();
shape.shape = new Int2[width*width];
// Create an array containing all integer points within a width*width square
int i = 0;
for (int x = -width/2; x <= width/2; x++) {
for (int z = -width/2; z <= width/2; z++) {
shape.shape[i] = new Int2(x, z);
i++;
}
}
return shape;
}
public bool CanTraverse (Path path, GraphNode node) {
GridNodeBase gridNode = node as GridNodeBase;
// Don't do anything special for non-grid nodes
if (gridNode == null) return DefaultITraversalProvider.CanTraverse(path, node);
int x0 = gridNode.XCoordinateInGrid;
int z0 = gridNode.ZCoordinateInGrid;
var grid = gridNode.Graph as GridGraph;
// Iterate through all the nodes in the shape around the current node
// and check if those nodes are also traversable.
for (int i = 0; i < shape.Length; i++) {
var inShapeNode = grid.GetNode(x0 + shape[i].x, z0 + shape[i].y);
if (inShapeNode == null || !innerProvider.CanTraverse(path, inShapeNode)) return false;
}
return true;
}
public bool CanTraverse (Path path, GraphNode from, GraphNode to) {
return CanTraverse(path, to);
}
public uint GetTraversalCost (Path path, GraphNode node) {
// Use the default traversal cost.
// Optionally this could be modified to e.g taking the average of the costs inside the shape.
return DefaultITraversalProvider.GetTraversalCost(path, node);
}
// This can be omitted in Unity 2021.3 and newer because a default implementation (returning true) can be used there.
public bool filterDiagonalGridConnections {
get {
return true;
}
}
}
This is almost the same as in the tutorial, but with a tiny change to the line highlighted in the image below:
One thing to note is that the GridShapeTraversalProvider only supports odd agent sizes. Other sizes will work too, but the path will no longer be centered on the character. You can adjust the path as a post-processing step, however. But you’ll have to write some code for that.
Hi Aron, just to clarify, I have my code working fine with both odd square and non-square shapes, I just thought to mention this:
My Traversal provider was more or less the default one from your examples but then I realized that the OverlappingNodesCost method you have is using the DefaultITraversalProvider.CanTraverse. Does that ever work? The default one isn’t checking BlockManager and I have to say it was the last piece of the puzzle for my solution.
My point was that since this example was for grids, does the DefaultITraversalProvider provide any help in your example there? Shouldn’t it check the BlockManager instead?
It was mostly a point for your docs as I’ve got my part working fine 
The BlockManager is only introduced in the turn based tutorial, and not really referenced anywhere else.
The tutorial on large characters has no reason to reference it? Or am I misunderstanding you?
Also. OverlappingNodesCost
is not something that exists in my package.