Hey,
What I want to do is preview the possible path character might take in a point and click game as player hovers the mouse around the terrain but before movement starts. Similar to path preview in Divinity OS2.
What is the closest possible approximation I can get of the actual path RichAI will take? I know it modifies the path to get smoother movement and I want the path preview to reflect actual path taken when character moves.
This only happens in turn-based mode, so there is no possibility of other characters moving at the same time altering path. Or dynamic obstacles etc.
I have been trying to figure out the same thing and I think I have a solution that works with some help from MetalStorm Games on YouTube for the Line Render: https://www.youtube.com/watch?v=dTlSk9cP7TA&ab_channel=MetalStormGames
I have not used the RichAI path, but I would imagine this would still be applicable since it uses the Seeker.
-
Add the LineRender component to the object you want to have to render your lines. This could be each character or a manager that has access to the characters.
-
Create a variable for the LineRender and make sure to assign the LineRender on Awake
private LineRenderer lineRenderer;
private void Awake()
{
lineRenderer = GetComponent();
}
- Set the LineRender parameters you want on Start. This will make sure you can see the line.
private void Start()
{
lineRenderer.startWidth = 0.15f;
lineRenderer.endWidth = 0.15f;
lineRenderer.positionCount = 0;
}
- Create a method if you haven’t already to find where the mouse position is pointing at in the world and store it in a Vector3 variable. The below method is using the new input system so make sure to adjust your script accordingly to use either your input system or the old Unity input system to get a ScreenPointToRay. There are lots of helpful guides and videos out there on how to do that.
private Vector3 mousePositon;
Ray ray = mainCamera.ScreenPointToRay(playerInput.actions[“MousePosition”].ReadValue());
RaycastHit hit;
if (Physics.Raycast(ray, out hit))
{
mousePosition = hit.point;
}
- Create a DrawPath method and check that you have a character selected so you don’t get any null references. This character should also have a Seeker on it and it should be accessible.
private void DrawnPath()
{
if(selectedCharacter == null) return;
}
- Create an OnPathComplete method if you don’t already have one that takes in a Path variable
private void OnPathComplete (Path p)
{
}
- Put the DrawPath method in Update. This is where you may want to set up a timer so this method doesn’t run every frame but instead every fraction of a second. It is up to you if you see performance hits or not.
private void Update()
{
DrawnPath();
}
- In the DrawPath method, call the StartPath method on your selected characters Seeker and set the parameters to the selected characters’ current position, and mousePosition, and the OnPathComplete
private void DrawnPath()
{
if(selectedCharacter == null) return;
selectedCharacter.seeker.StartPath(selectedCharacter.transform.position, mousePosition, OnPathComplete);
}
- In on PathComplete put the logic for rendering your line (more info on this in the video above)
private void OnPathComplete (Path p)
{
lineRenderer.positionCount = p.vectorPath.Count;
if(p.vectorPath.Count < 2) return;
lineRenderer.SetPosition(0, selectedCharacters[0].transform.position);
if(p.vectorPath.Count < 2) return;
for (int i = 0; i < p.vectorPath.Count; i++)
{
lineRenderer.SetPosition(i, p.vectorPath[i]);
}
}
- Test it out and then adapt it to your project. You will likely want a way to clear the LineRender once the selected character moves as well as turn off the DrawPath method when it is not needed but this should get you started
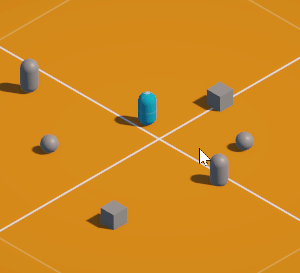
2 Likes