I’m using the 4.3.60 beta of the package and struggling a bit with setting up our player character as a navmesh obstacle. It seems that RVOObstacle was deprecated in the beta package they’re using and I’m not sure what the replacement is. Docs don’t mention obstacles and the comment in RVOObstacle.cs is quite vague.
“// Deprecated: This component is deprecated. Local avoidance colliders never worked particularly well and the performance was poor. Modify the graphs instead so that pathfinding takes obstacles into account.”
The desired behaviour is that our AI doesn’t intersect with the player. We’re using RichAi on a basic navmesh graph. We tried using cutting on a recast graph but that was costing us 3.5ms + 100KB of garbage for every cut as the player moved around. We’ve also converted to fixed navmesh graphs because the memory cost and load time for recast was astronomical in our case.
I saw a few other topics on this but none seemed to answer my use case.
Hi
If you want your AI to avoid the player, then the best solution is to attach an RVOController to the player character, but override its velocity every frame. See RVOController - A* Pathfinding Project
Could cached startup perhaps help you in this case? See A* Pathfinding Project
This doesn’t seem to do anything. I’ve attached an RVO controller and am setting it’s velocity to zero in every frame as you suggested.
This is our character setup
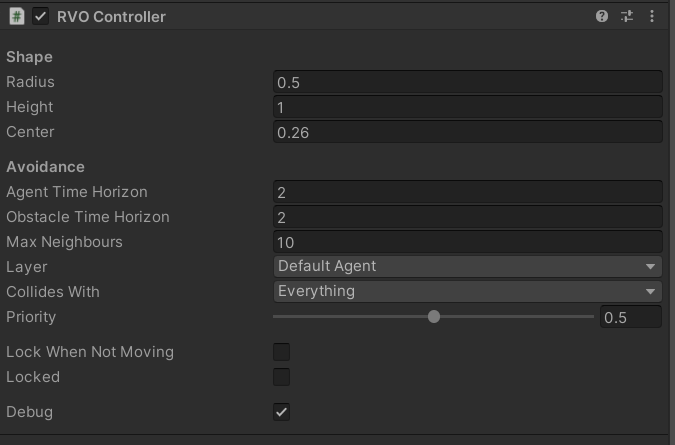
Our AI
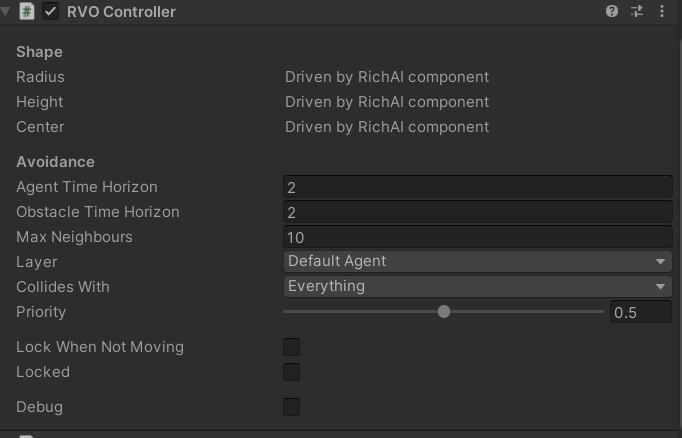
Could cached startup perhaps help you in this case? See Saving and Loading Graphs - A* Pathfinding Project
We tried this but loading was still quite slow. Switching to static navmeshes reduced our load time from around 30s to 2-3s. The memory impact is a big reason to do this also as we saved around 1.5GB just by switching graph type. Just exported as obj and used the same data.
That’s really strange. The recast graph is using literally the same data structures under the hood. The memory usage should be almost identical…
I did a deep memory profile there were something like 30 million more scripting objects in managed memory with recast compared to fixed navmesh
Just to make sure, here is a testing script for the functionality. I just tested it in an RVO scene and it worked just fine:
using Pathfinding.RVO;
using UnityEngine;
public class PlayerController : MonoBehaviour {
RVOController rvo;
public float speed = 5;
void Awake() {
rvo = GetComponent<RVOController>();
}
void Update() {
var dx = Input.GetAxis("Horizontal");
var dy = Input.GetAxis("Vertical");
var v = new Vector3(dx, 0, dy) * speed;
rvo.velocity = v;
transform.position += v * Time.deltaTime;
}
}
Was this after loading from a cache or not?
Ok so I tried the cache with the basic navmeshes (assuming this would be a nice load time boost on top) but hadn’t tried it properly on the Recast meshes. I’ve tried this on recast and am now seeing exactly the same memory usage and loading times. Thanks for the tip on that!
Regarding the obstacle stuff, I’ve realised it’s more complicated than I initially thought. I’m optimising someone else’s pathfinding and have just realised their AI actually does it’s own thing most of the time, only seemingly using your A* for path checking and some other bits. So it doesn’t look like any of the avoidance between AI is working either. I will investigate this further and get back to you!
1 Like