Okay so I found the issue, now I dont know if its a bug or intended, but seems odd.
Basically the ersion of adjacent colliders take priority over untraversable nodes. In my mind it should be the other way around? Because atm if I place an obstacle with a collider next to an obstacle that is set to untraversable, part of that untraversable obstacle all of a sudden becomes walkable because of the erosion of the adjacent collider obstacle.(To be clear, I use both collider based obstacles, and tag based, tag based are only for obstacles that have different rules for different AI, for example water)
On top of that, when I place an collider obstacle during runtime and call the code below, several untraversable nodes are reset, even ones not adjacent to the erosion.
public void UpdateGridGraph(Bounds bounds)
{
var guo = new GraphUpdateObject(bounds);
guo.updatePhysics = true;
AstarPath.active.UpdateGraphs(guo);
}
This is what the GraphUpdateScene obstacle looks like for my tag based obstacle:
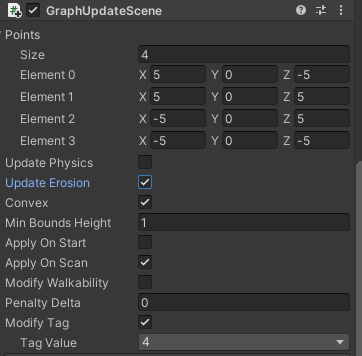
So basically two big problems for me:
- Erosion overwrites untraversable nodes
-
UpdateGraphs(guo)
when removing a collider obstacle resets several untraversable nodes that are not within the bounds of that obstacle.
Here are some images for clarity:
Red obstacles are untraversable, purple obstacle uses a collider, so is completely unwalkable:
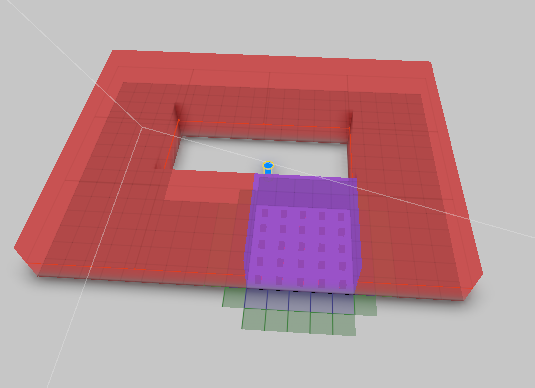
The above but without meshes for clarity, as you can see erosion takes priority:
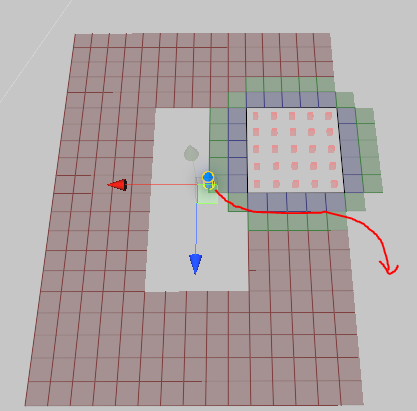
Here is a shot AFTER I set the collider obstacle as a trigger and call UpdateGraphs(guo)
, as you can see it does not look good, in my game this scenario is typical when destroying a building:
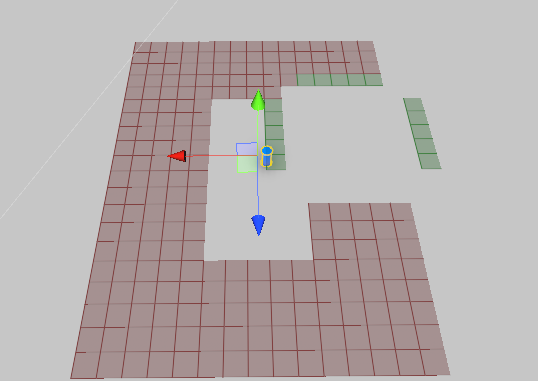
Another scenario with erosion off:
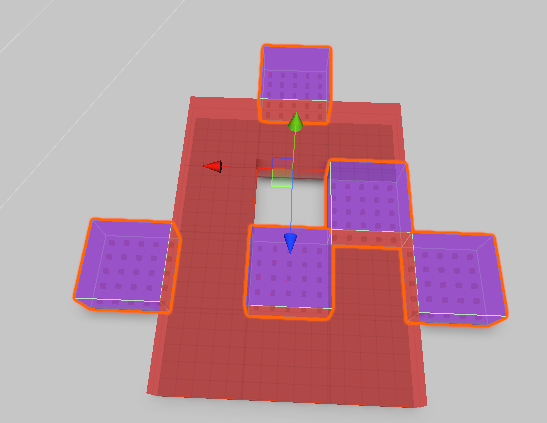
Here is a shot AFTER I set the collider obstacle as a trigger and call UpdateGraphs(guo)
, as you can see it still effects adjacent untraversable nodes, in my game this scenario is typical when destroying a building: